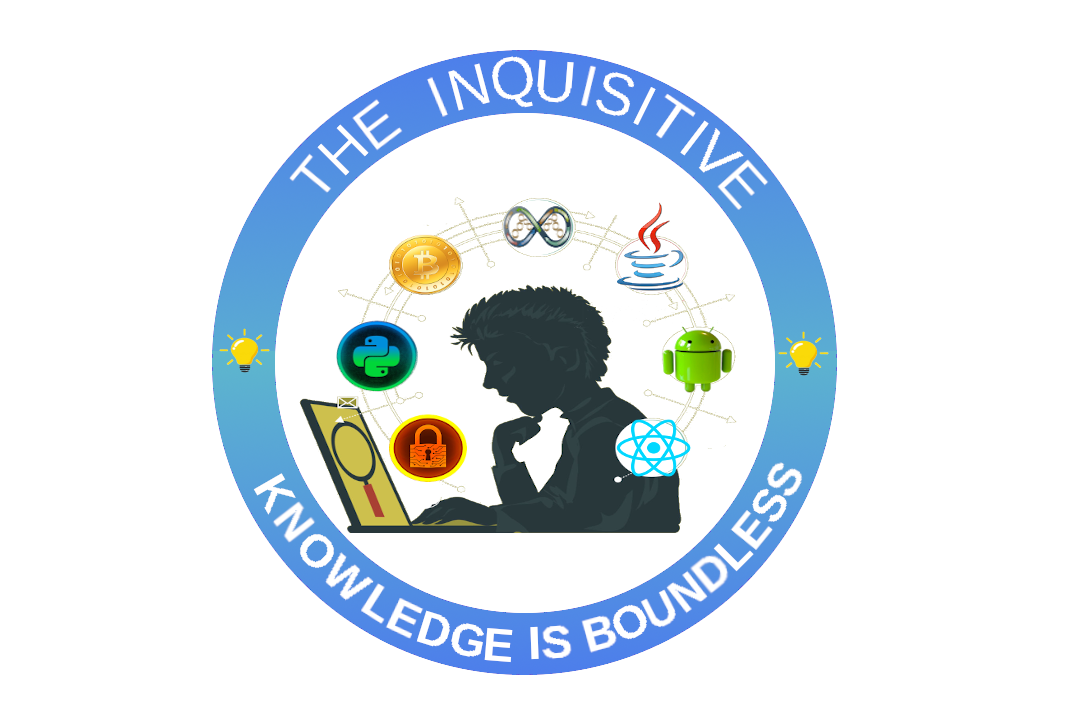
page 1 out of 4
page 1 out of 4
Question 1
Question 2
Question 3
Question 4
Question 5
Question 6
Question 7
Question 8
Node find(Node node, int key) { if (node == null) { node = new Node(key); return node; } if (key < node.key) node.left = find(node.left, key); else if (key > node.key) node.right = find(node.right, key); return node; }
Question 9
public Node find(Node node, int key) { if (node==null || node.key==key) return node; if (node.key > key) return find(node.left, key); return find(node.right, key); }
Question 10
Node find(Node node, int key) { if (node == null) return node; if (key < node.key) node.left = find(node.left, key); else if (key > node.key) node.right = find(node.right, key); else { if (node.left == null) return node.right; else if (node.right == null) return node.left; node.key = minValue(node.right); node.right = find(node.right, node.key); } return node; }
page 1 out of 4
page 1 out of 4
Oops!!
To view the solution need to Login