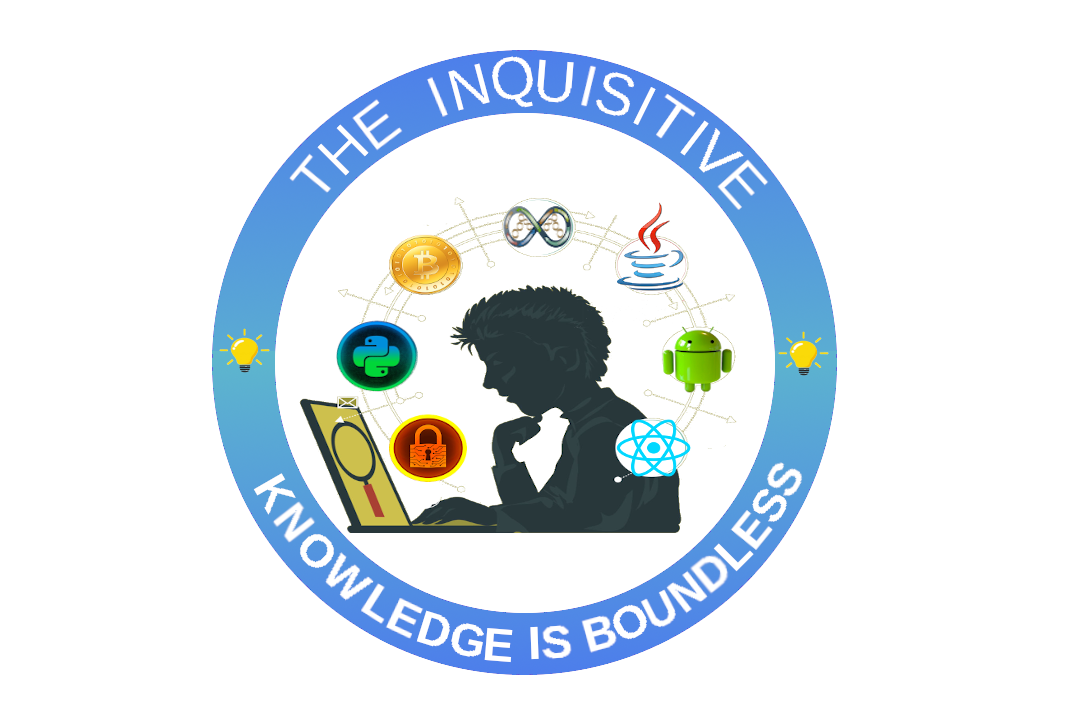
page 1 out of 3
page 1 out of 3
Question 1
#include<stdio.h> int main() { static char *s[] = {"black", "white", "pink", "violet"}; char **ptr[] = {s+3, s+2, s+1, s}, ***p; p = ptr; ++p; printf("%s", **p+1); return 0; }
Question 2
void print(int *ptr) { *ptr = 65; } int main() { int x = 60; print(&x); printf("%d", x); return 0; }
Question 3
Question 4
#include <stdio.h> int main() { int a = 10; void *p = &a; int *ptr = p; printf(“%u”,*ptr); return 0; }
Question 5
int main() { char *a = NULL; char *b = 0; if (a) printf(" A "); else printf("null A"); if (!b) printf("B\n"); else printf(" null B\n"); }
Question 6
int main() { int i = 10; void *p = &i; printf("%f\n", *(float*)p); return 0; }
Question 7
int *f(); int main() { int *p = f(); printf("%d\n", *p); } int *f() { int *j = (int*)malloc(sizeof(int)); *j = 10; return j; }
Question 8
int *f(); int main() { int *p = f(); printf("%d\n", *p); } int *f() { int j = 10; return &j; }
Question 9
Question 10
page 1 out of 3
page 1 out of 3
Oops!!
To view the solution need to Login